![Creative The name of the picture]()
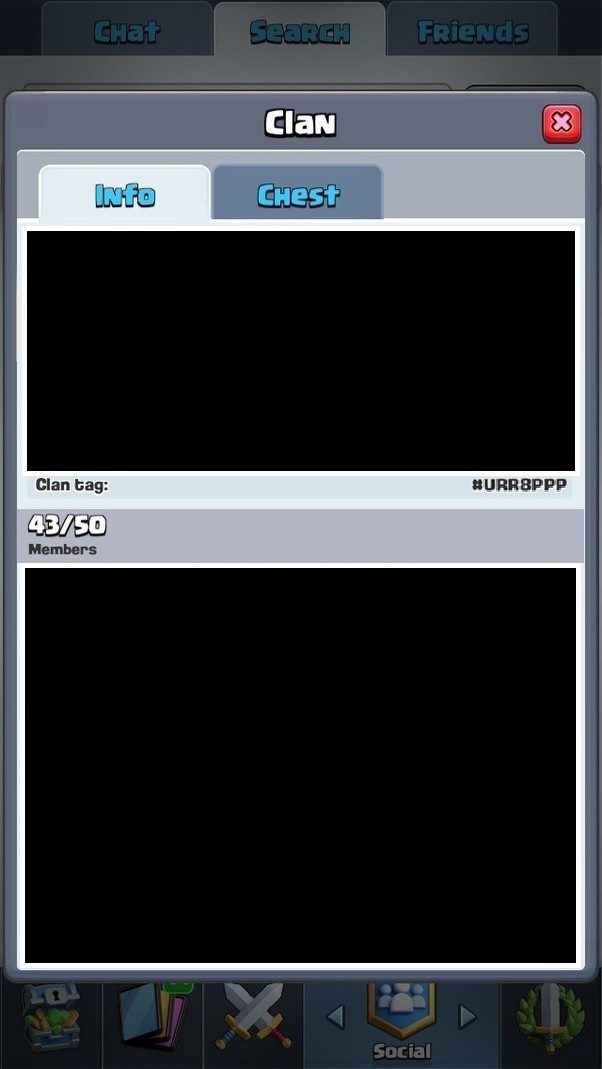
Clash Royale CLAN TAG#URR8PPP
Call method within method in reactjs
I want to call a method inside another method like this, but it is never called.
Button:
<span onClick={this.firstMethod()}>Click me</span>
Component:
class App extends Component {
constructor(props) {
super(props);
this.state = {..};
}
firstMethod = () => (event) => {
console.log("button clicked")
this.secondMethod();
}
secondMethod = () => (event) => {
console.log("this is never called!")
}
render() {..}
}
The first method is called, but not the second one. I tried to add to the constructor
this.secondMethod = this.secondMethod.bind(this);
which is recommended in all the other solutions but nothing seems to work for me. How can I call the second method correctly?
6 Answers
6
You van use
<span onClick={() => this.firstMethod(parameters...)}>Click me</span>
Instead of firstMethod = () => (event)
try firstMethod = (event) =>
and instead of secondMethod = () => (event) => {
try secondMethod = (event) => {
firstMethod = () => (event)
firstMethod = (event) =>
secondMethod = () => (event) => {
secondMethod = (event) => {
onClick
<span onClick={this.firstMethod}>Click me</span>
The bad news is that you can't bind
arrow functions because they're lexically bound. See:
bind
Can you bind arrow functions?
The good news is that "lexical binding" means that they should already have App as their this
, i.e. it should would without explicitly binding. You'd likely redefining them as undefined, or some other odd thing by treating them this way in the constructor.
this
There are two problems here.
First one: You are defining your functions wrong.
firstMethod = () => (event) => {
console.log("button clicked")
this.secondMethod();
}
Like this, you are returning another function from your function. So, it should be:
firstMethod = ( event ) => {
console.log("button clicked")
this.secondMethod();
}
Second: You are not using onClick
handler, instead invoking the function immediately.
onClick
<span onClick={this.firstMethod()}>Click me</span>
So, this should be:
<span onClick={() => this.firstMethod()}>Click me</span>
If you use the first version, yours, when component renders first time your function runs immediately, but click does not work. onClick
needs a handler.
onClick
Lastly, if you define your functions as an arrow one, you don't need to .bind
them.
.bind
Here is the working code.
class App extends React.Component {
firstMethod = () => {
console.log("button clicked")
this.secondMethod();
}
secondMethod = () =>
console.log("this is never called!")
render() {
return(
<span onClick={() => this.firstMethod()}>Click me</span>
)
}
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
Try this, it works for me.
firstMethod = () => {
console.log("click handler for button is provided")
return (event) => this.secondMethod(event);
}
secondMethod = (event) => {
console.log("This is being called with", event);
}
Your second method returns a new function, which is redundant.
Also, your second method can be not bound, since the first method has the context already.
secondMethod = () => (event) => { ... }
should be secondMethod(evnt) { ... }
secondMethod = () => (event) => { ... }
secondMethod(evnt) { ... }
Here is the working and optimized example https://codesandbox.io/s/pkl90rqmyj
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
and
onClick
event/prop should not be called with '()'. it should look like this:<span onClick={this.firstMethod}>Click me</span>
– Danko
5 mins ago