![Creative The name of the picture]()
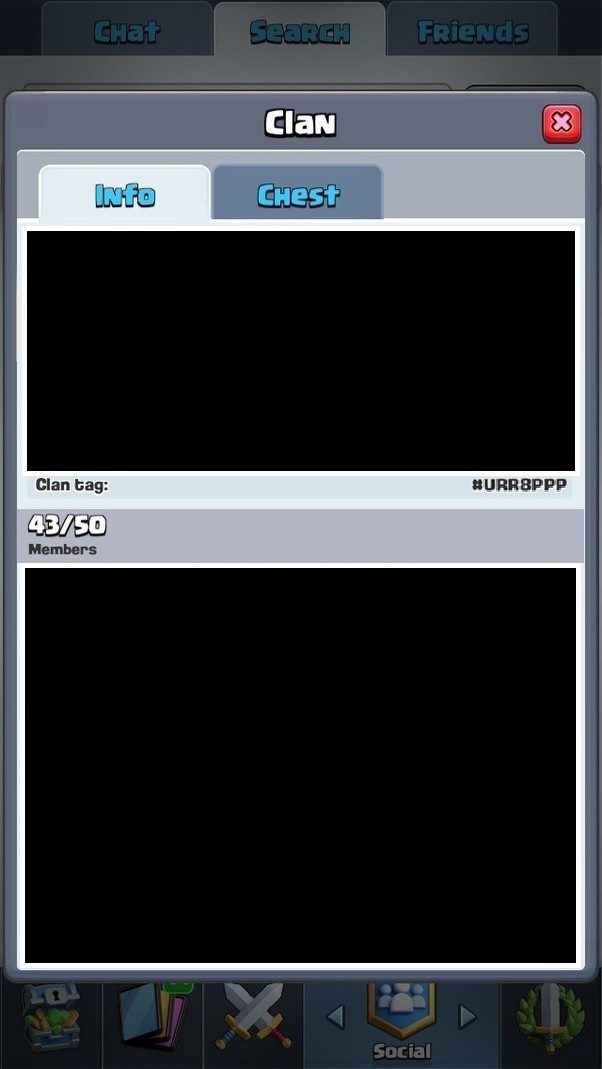
Clash Royale CLAN TAG#URR8PPP
Handle table inside another HTML table without interfering with JAVASCRIPT
I have a table with id = mytabla, which inside contains several data, and in the last row a field called retentions that should show another table inside, in this I have no problems, thus:
<table id="mytabla" class="table table-sm table-striped">
<tbody>
<tr>
<th id="#">#</th>
<th id="Documento" class="tb-gra">Documento</th>
<th id="Fecha">Fecha(emision)</th>
<th id="retenciones">Retenciones</th>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>
<table><tbody>
<tr>
<td>IVA</td>
<td>3</td>
<td>120.00</td>
<td>100.0</td>
<td>120.00</td>
</tr>
</tbody></table>
</td>
</tr>
</tbody>
</table>
in javascript I want to select the table and count the rows, but also tell me those of the second table, as I do so that the second table does not count or do not take it into account, I use this code:
var table = document.getElementById("mytabla");
var fila=document.getElementById("mytabla").getElementsByTagName('tr');
var row=table.insertRow(parseInt(fila.length);
var cantidaddefilas=fila.length;
pero me esta contando las de la segunda tabla, como hago para que no cuente esas.
4 Answers
4
You should use a querySelector
.
querySelector
document.querySelectorAll('#mytabla > tbody > tr');//selects all table rows whose parent is a tbody that is a child of #mytabla
<table id="mytabla" class="table table-sm table-striped">
<tbody>
<tr>
<th id="#">#</th>
<th id="Documento" class="tb-gra">Documento</th>
<th id="Fecha">Fecha(emision)</th>
<th id="retenciones">Retenciones</th>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>
<table><tbody>
<tr>
<td>IVA</td>
<td>3</td>
<td>120.00</td>
<td>100.0</td>
<td>120.00</td>
</tr>
</tbody></table>
</td>
</tr>
</tbody>
</table>
<script>
console.log(document.querySelectorAll('#mytabla > tbody > tr').length);
</script>
In the case of a table
, you can actually just get the length of its rows
attribute https://www.w3schools.com/jsref/coll_table_rows.asp
table
rows
var cantidaddefilas = document.getElementById('mytabla').rows.length
Im not sure that I understand question. You want to count all rows, except rows in inner table?
Then, instead of
var fila=document.getElementById("mytabla").getElementsByTagName('tr');
use
var fila=document.querySelectorAll("#mytabla > tbody > tr");
Also, you don't need to parseInt(fila.length)
. Length property is a number, so fila.length
is enough.
parseInt(fila.length)
fila.length
You can use querySelectorAll
like in the example snippet below.
querySelectorAll
var fila=document.querySelectorAll("#mytabla > tbody > tr");
alert('Number of rows: '+fila.length);
<table id="mytabla" class="table table-sm table-striped">
<tbody>
<tr>
<th id="#">#</th>
<th id="Documento" class="tb-gra">Documento</th>
<th id="Fecha">Fecha(emision)</th>
<th id="retenciones">Retenciones</th>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>
<table><tbody>
<tr>
<td>IVA</td>
<td>3</td>
<td>120.00</td>
<td>100.0</td>
<td>120.00</td>
</tr>
</tbody></table>
</td>
</tr>
</tbody>
</table>
OR you could use getElementsByClassName
after changing your HTML
a little. Check this example also.
getElementsByClassName
HTML
var fila = document.getElementsByClassName('mytabla');
alert('Number of rows: '+fila.length);
<table id="mytabla" class="table table-sm table-striped">
<tbody>
<tr class="mytabla">
<th id="#">#</th>
<th id="Documento" class="tb-gra">Documento</th>
<th id="Fecha">Fecha(emision)</th>
<th id="retenciones">Retenciones</th>
</tr>
<tr class="mytabla">
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr class="mytabla">
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr class="mytabla">
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>
<table><tbody>
<tr>
<td>IVA</td>
<td>3</td>
<td>120.00</td>
<td>100.0</td>
<td>120.00</td>
</tr>
</tbody></table>
</td>
</tr>
</tbody>
</table>
in case you don't want to count header row change your HTML
by using a thead
element.
HTML
thead
var fila=document.querySelectorAll("#mytabla > tbody > tr");
alert('Number of rows: '+fila.length);
<table id="mytabla" class="table table-sm table-striped">
<thead>
<tr>
<th id="#">#</th>
<th id="Documento" class="tb-gra">Documento</th>
<th id="Fecha">Fecha(emision)</th>
<th id="retenciones">Retenciones</th>
</tr>
</thead>
<tbody>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>SIN RETENCION</td>
</tr>
<tr>
<td class="tb-neg">1</td>
<td class="tb-gra">FACTURA</td>
<td style="width:64pt">04/06/2018</td>
<td>
<table><tbody>
<tr>
<td>IVA</td>
<td>3</td>
<td>120.00</td>
<td>100.0</td>
<td>120.00</td>
</tr>
</tbody></table>
</td>
</tr>
</tbody>
</table>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You forgot to translate the last line into english. I would edit it but I don't want to change the meaning of what you want.
– chevybow
28 mins ago