Grab Value from HTML Table jQuery
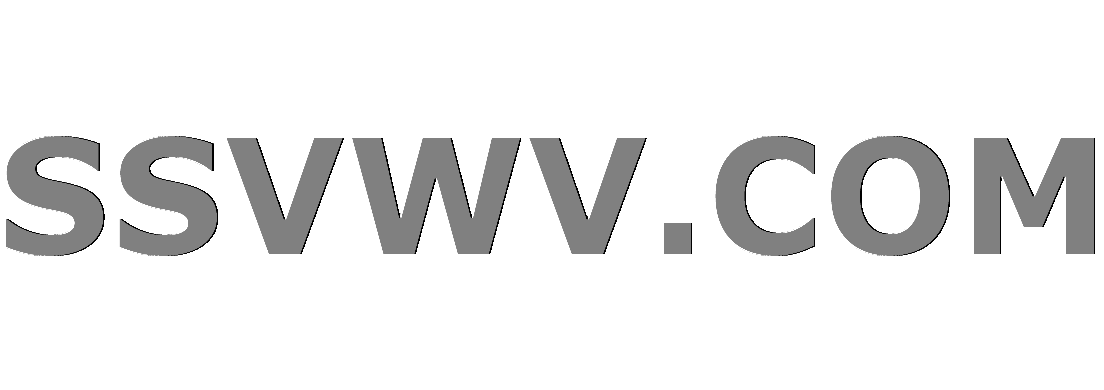
Multi tool use
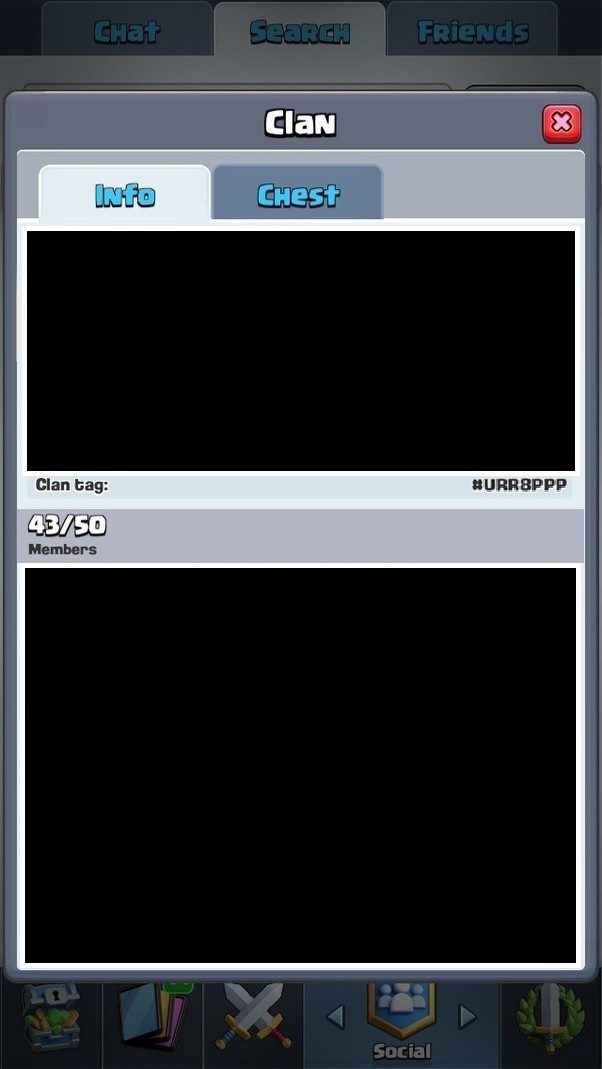

Grab Value from HTML Table jQuery
I have an HTML table with the following values:
<tr class="tuker1">
<td class="tuker-lft1">
Username
</td>
<td class="tuker-rght1" onclick="awardRoute()">
<a href="#">
AWARD ROUTE
</a>
</td>
</tr>
When I click the AWARD ROUTE link I would like the value inside "tuker-lft1" to go into the function variable.
awardRoute() {
var username = "";
}
Any ideas on how I can accomplish this?
6 Answers
6
Assuming that you only have one element on the page with the class tuker-lft1
:
tuker-lft1
function awardRoute() {
var username = $('.tuker-lft1').text();
}
If you want the text of the previous cell (i.e. the cell to the left), you can use sibling tree traversal functions:
function awardRoute() {
var username = $(this).prev('td').text();
}
$('.tuker-rght1').click(awardRoute);
If you want the text in the cell that has the class tuker-lft1
within the same row as the cell you're clicking on, regardless of where the cell is, use parent/child tree traversal functions:
tuker-lft1
function awardRoute() {
var username = $(this).parent().children('.tuker-lft1').text();
}
$('.tuker-rght1').click(awardRoute);
Note that in the last two examples, you should remove the onclick attribute from your HTML.
this
isn't what you think it is– charlietfl
Jul 20 '14 at 23:08
this
@charlietfl Thanks, fixed.
– rink.attendant.6
Jul 20 '14 at 23:17
the way you had it have to pass
this
as argument from markup, then use awardRoute(elem){ $(elem).prev()...}
– charlietfl
Jul 20 '14 at 23:20
this
awardRoute(elem){ $(elem).prev()...}
You can grab it like this:
<td class="tuker-rght1" onclick="$(this).parents('tr').find('td:eq(0)').html()"><a href="#"> AWARD ROUTE </a> </td>
<td class="tuker1">
<td class="tuker-lft1"> Username </td>
<td class="tuker-rght1"> <a href="#"> AWARD ROUTE </a> </td>
</td>
$(function(){
$('.tuker-rght1').on('click', function(e){
e.preventDefault();
var username = $(this).prev().html();
console.log(username);
});
});
Use this:
var username = $(this).closest('tr').find('.tuker-lft1').text();
Please refer the fiddle
Markup:
<table>
<tr>
<td>
Username
</td>
<td class="award">
Award
</td>
</tr>
</table>
JS:
var awards = .slice.call(document.querySelectorAll('.award'));
awards.forEach(function(award){
award.addEventListener('click', function(e){
var parentNode = this.parentNode;
var username = parentNode.querySelector('td').innerHTML;
alert(username);
});
});
var username = $(".tuker-lft1").val()
.val()
? I think that is only for inputs– dmullings
Jul 20 '14 at 22:59
.val()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You need plain js or a jQuery solution would be fine?
– Marios Fakiolas
Jul 20 '14 at 22:57