![Creative The name of the picture]()
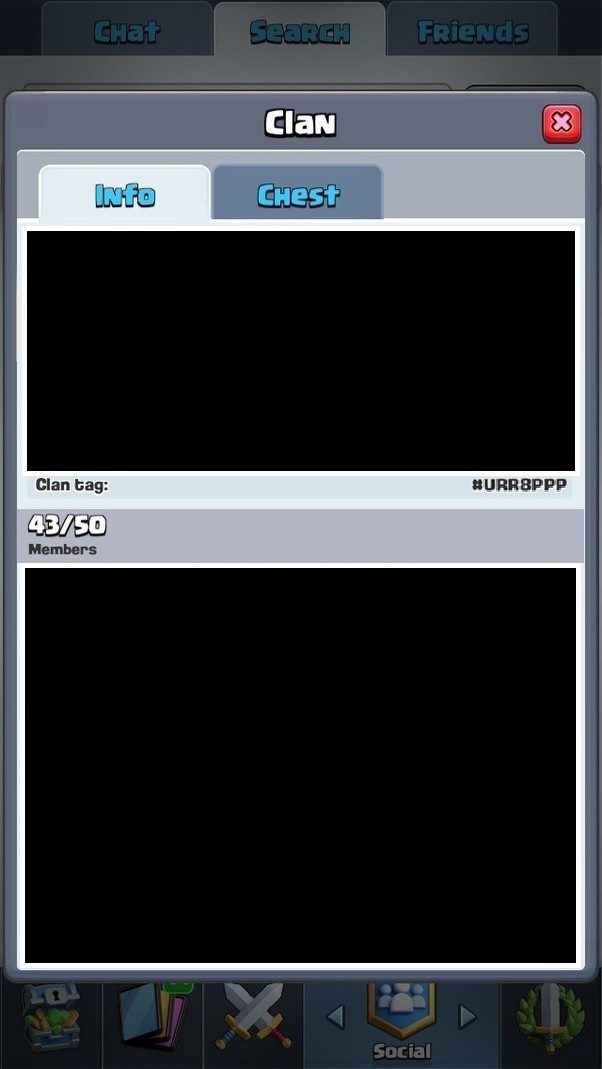
Clash Royale CLAN TAG#URR8PPP
Counting white pixels between 2 points in an image
I found starting and ending points of this thick white line and these are [234, 2]
and [134, 397]
. Now I want to calculate number of white pixels that are lying between these 2 points on a straight way. My purpose is to calculate the number of white pixels not on the thick line. I want to count white pixels that are lying on the line of width of 1 pixel. I'm very new to Python. I spent whole day in finding my solution but failed to find any way. How can I find that?
[234, 2]
[134, 397]

My effort to find the approximate starting and ending points is:
for y in range(height):
for x in range(width):
....
....
print(p1," , ",p2)
Now I'm facing trouble in next step. i.e. counting the number of white pixels between these 2 points.
2 Answers
2
According to the Bresenham line drawing algorithm, there are exactly
max(abs(p1.x - p2.x), abs(p1.y - p2.y)) - 1
pixels in between points p1
and p2
(not counting the pixels p1
or p2
).
p1
p2
p1
p2
This line is a 1-pixel thick line.
Note that the number of pixels does not correspond to the Euclidean distance between the points, but to the L-infinity distance, also called max-norm, chessboard distance or Chebyshev distance.
Edit: The updated question is totally different. The above will be the upper bound.
To count the actual number of white pixels on the line between the two points, you need to draw that line. The Wikipedia link above gives details on the algorithm to draw lines. Here is a very simple implementation in MATLAB, should be easy to translate to Python. Though instead of actually drawing the line, you would read the pixel values, and count the ones that are white.
@Ben Jones suggested a Python implementation of Bresenham's algorithm. It needn't be that complicated. Here is a simple Python script that finds all pixels on the straight line between the two points (translated from the MATLAB version linked above):
p1 = np.array([0,0])
p2 = np.array([7,4]) % Two example points.
p = p1
d = p2-p1
N = np.max(np.abs(d))
s = d/N
print(np.rint(p).astype('int'))
for ii in range(0,N):
p = p+s;
print(np.rint(p).astype('int'))
This produces the output:
[0 0]
[1 1]
[2 1]
[3 2]
[4 2]
[5 3]
[6 3]
[7 4]
Now all that's left is reading the pixel values at these coordinates, and determining whether they're white or not.
If you are using a line algorithm like Bresenham's, and both points are on the thick white line as you have described, then the number of white pixels between the two points will actually be something like:
num_white_pixels = max(abs(pt0[0] - pt1[0]), abs(pt0[1] - pt1[1]))
Because, presumably the entire line will fall in the white region, and the number of pixels that make up a line of width 1 is just max( dx, dy )
, give or take one pixel, depending on whether you want to include the end points.
max( dx, dy )
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You need to decide which line drawing algorithm you want to rasterize the line between two points. Once you determine that, this should be fairly simple.
– Ben Jones
yesterday