![Creative The name of the picture]()
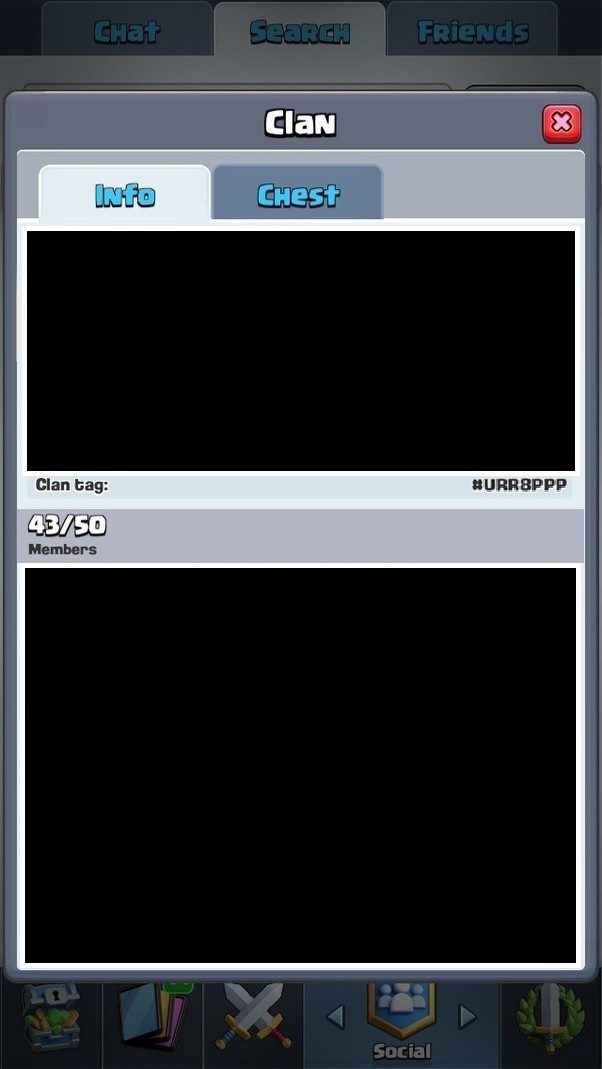
Clash Royale CLAN TAG#URR8PPP
How to implement lazy load for images returned from a server in ES5?
On one page, I'm trying to lazy load potentially hundreds of images that are returned from my server as blobs. I've tried converting them to base64 data uris then using some lazy loading techniques, but understandably that didn't work, since the data uri is already in the html, which means the browser still needs to load the image data even if it's not going to display it right away.
Is there some way to lazy load dynamic images returned by a server as blobs? Or some way to convert them to use remote image urls then use typical lazy loading that way?
Thanks for any help.
1 Answer
1
ES5 approach : XMLHttpRequest
In this scenario you get an arraybuffer
from the ajax request, that must be converted into a base64
string, before being useful. (IE10+ Compatible Method)
arraybuffer
base64
let myImg = document.getElementById('myImg');
let url = "https://placekitten.com/200/300";
let request = new XMLHttpRequest();
request.open ("GET", url, true);
request.responseType = "arraybuffer";
request.send (null);
request.onreadystatechange= function(response){
if (request.readyState === 4) {
if (request.status === 200) {
// convert received arrayBuffer into base64 encoded string
var imgBase64 = btoa(String.fromCharCode.apply(null, new Uint8Array(request.response)));
// assign base64 encoded image to image source
myImg.src= 'data:image/jpeg;base64,' + imgBase64;
}
}
}
<img id="myImg" />
Note: CORS must be taken in consideration, when retrieving images from external domains.
ES6 approach : Fetch
You can use fetch
to retrieve your images, and inject them in the DOM as soon as you receive them...
fetch
let myImage = document.getElementById('myImg');
let url = "https://placekitten.com/200/300";
fetch(url)
.then( response=> {
// if response is OK, covert it into a blob
if(response.ok) return response.blob();
else throw new Error('Response not OK');
})
.then(myBlob=>{
// Assign it to the image src using createObjectURL
myImage.src = URL.createObjectURL(myBlob);
})
.catch(function(error){
// hadle errors
console.log(error.message);
});
<img id="myImg" />
You can get more details about Fetch and createObjectURL in the Mozilla Developer webpage.
Previous code can be easily converted into reusable functions, suitable for generic scenarios.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
If you have received them already, you do not have to load them again. Please add a Minimal, Complete, and Verifiable example to get more help.
– Kosh Very
yesterday