![Creative The name of the picture]()
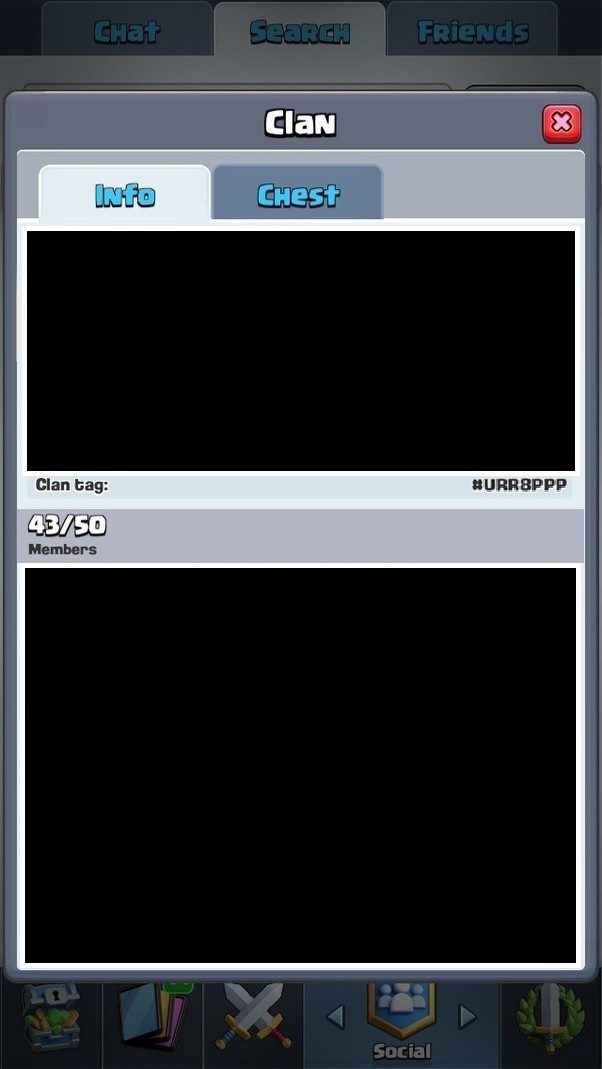
Clash Royale CLAN TAG#URR8PPP
ERROR TypeError: Cannot set property 'paginator' of undefined
I am creating a table using table Angular material
for reference I am using this example https://material.angular.io/components/table/examples
Here is what I have so far:
HTML
<mat-form-field>
<input matInput (keyup)="applyFilter($event.target.value)" placeholder="Filter">
</mat-form-field>
<div class="mat-elevation-z8">
<table mat-table [dataSource]="dataSource" matSort>
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Name </th>
<td mat-cell *matCellDef="let row"> {{row.name}} </td>
</ng-container>
<ng-container matColumnDef="id">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Id </th>
<td mat-cell *matCellDef="let row"> {{row.id}} </td>
</ng-container>
<ng-container matColumnDef="release">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Release</th>
<td mat-cell *matCellDef="let row"> {{row.first_air_date}} </td>
</ng-container>
<ng-container matColumnDef="description">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Description </th>
<td mat-cell *matCellDef="let row" [style.color]="row.color"> {{row.overview}} </td>
</ng-container>
<tr mat-header-row *matHeaderRowDef="displayedColumns"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColumns;">
</tr>
</table>
<mat-paginator [pageSizeOptions]="[5, 10, 25, 100]"></mat-paginator>
</div>
Component .ts
import { Component, ViewChild, OnInit } from '@angular/core';
import { MoviesService } from '../movies.service';
import { MatPaginator, MatSort, MatTableDataSource } from '@angular/material';
import { DatatableComponent } from '@swimlane/ngx-datatable';
@Component({
selector: 'app-tv-shows',
templateUrl: './tv-shows.component.html',
styleUrls: ['./tv-shows.component.scss']
})
export class TvShowsComponent implements OnInit {
displayedColumns: string = ['name', 'id', 'release', 'description'];
dataSource: any;
@ViewChild(DatatableComponent) table: DatatableComponent;
constructor(private moviesService: MoviesService) {
this.moviesService.getPopularTVShows().subscribe(res => {
this.dataSource = res.results;
});
}
@ViewChild(MatPaginator) paginator: MatPaginator;
@ViewChild(MatSort) sort: MatSort;
ngOnInit() {
this.dataSource.paginator = this.paginator;
this.dataSource.sort = this.sort;
}
applyFilter(filterValue: string) {
this.dataSource.filter = filterValue.trim().toLowerCase();
if (this.dataSource.paginator) {
this.dataSource.paginator.firstPage();
}
}
}
when I run my app data are displayed in a table perfectly but I get the following error :
ERROR TypeError: Cannot set property 'paginator' of undefined
So nothing works at all neither sorting , filtering or pagination
I need some help here:
What am I doing wrong in my codes above? Any help will be appreciated.
1 Answer
1
The error says that you're trying to assign this.dataSource.paginator
when this.dataSource
is still undefined
. Do the assignment after it was initalized. Also, when you want to use the paginator, sort or a filter, you have to use the MatTableDataSource
class for it. It's not enough to use raw data as your dataSource
:
this.dataSource.paginator
this.dataSource
undefined
MatTableDataSource
dataSource
ngOnInit() {
this.moviesService.getPopularTVShows().subscribe(res => {
this.dataSource = new MatTableDataSource(res.results);
^^^^^^^^^^^^^^^^^^
this.dataSource.paginator = this.paginator;
this.dataSource.sort = this.sort;
});
}
Cannot set property 'paginator' of undefined
this.dataSource.paginator
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I think the problem is this.paginator , this.sort are undefined at the time of invoke ngOnInit
– Muhammed Albarmawi
yesterday