![Creative The name of the picture]()
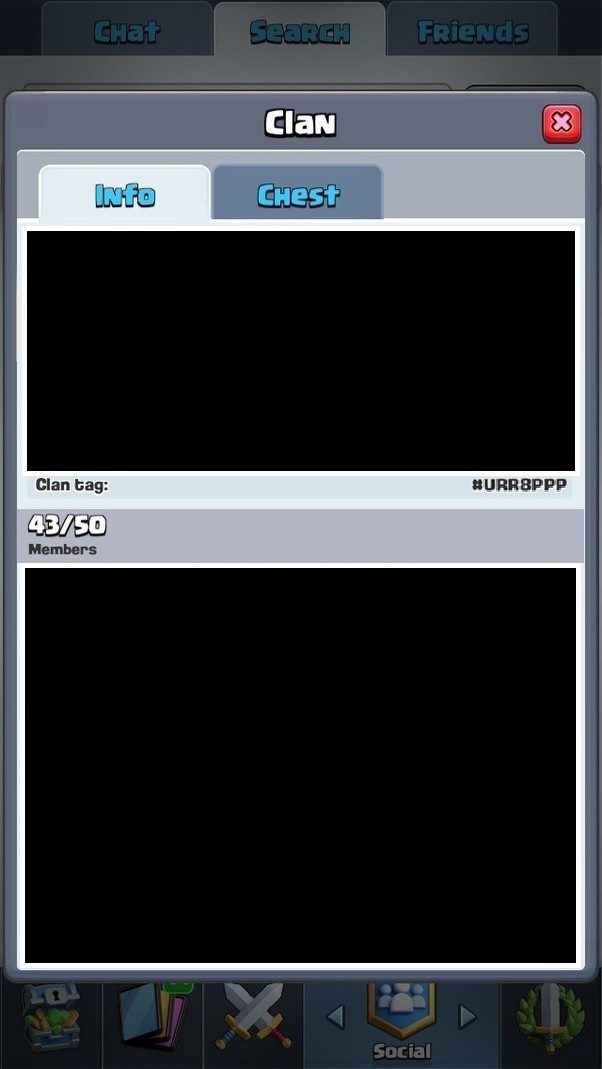
Clash Royale CLAN TAG#URR8PPP
How to pass a whole structure in and out of a function using an Arduino / C++
I'm pretty new to programming for Arduino, and am slightly confused about how I can use structures with functions.
Below is an example - I have 2 sets of data stored in a structure, struct1 - firstSet and secondSet. I want to take each of these sets, and apply the function to them, changing all values. I then want to print the new values to the console, and the numbers should grow larger with each iteration, firstSet twice as fast as secondSet.
This doesn't happen, and the initial values are printed over and over again. I'm not sure what I need to do to feed those values back out to their original structures.
I've been looking at adding a return line, and also changing void to something else (int and void*), but I really can't seem to work this out. Is it even possible? Alternatively is there a better way to do this? Thanks in advance!
#include "Arduino.h"
//The setup function is called once at startup of the sketch
struct struct1
{
int val1 = 1;
int val2 = 2;
int val3 = 3;
};
struct1 firstSet;
struct1 secondSet;
void func1(struct struct1)
{
struct1 dataSet;
dataSet.val1 = dataSet.val2;
dataSet.val2 = dataSet.val3;
dataSet.val3++;
}
void setup()
{
Serial.begin(9600);
while (! Serial);
Serial.println("Console Online");
}
void printAll()
{
Serial.println(firstSet.val1);
Serial.println(firstSet.val2);
Serial.println(firstSet.val3);
Serial.println("---");
Serial.println(secondSet.val1);
Serial.println(secondSet.val2);
Serial.println(secondSet.val3);
Serial.println("---END---");
}
// The loop function is called in an endless loop
void loop()
{
func1(firstSet);
func1(firstSet);
func1(secondSet);
printAll();
delay (1000);
}
func1
2 Answers
2
func1
is taking a struct struct1
, i.e. a copy of a struct as a parameter. As a result, changes made in the function are not visible in the calling code. Even then, you're not actually changing the parameter but a local variable.
func1
struct struct1
You need to declare the parameter as a reference to link the parameter to the variable in the calling function. Then you'll see the changes.
void func1(struct1 &dataSet)
{
dataSet.val1 = dataSet.val2;
dataSet.val2 = dataSet.val3;
dataSet.val3++;
}
I'm guessing a bit on the detail of what you want, but the essense of the answer is that your function must take a reference to the struct.
void func1(struct1& dataSet)
{
dataSet.val1 = dataSet.val2;
dataSet.val2 = dataSet.val3;
dataSet.val3++;
}
The &
makes dataSet
a reference. Your code was passing a copy of the structure (among other errors) which is why it could never alter the original structure.
&
dataSet
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
if i understood correctly, passing by reference is what you are looking for. get a good c++ book to learn the basics (stackoverflow.com/questions/388242/…)
– skeller
yesterday