![Creative The name of the picture]()
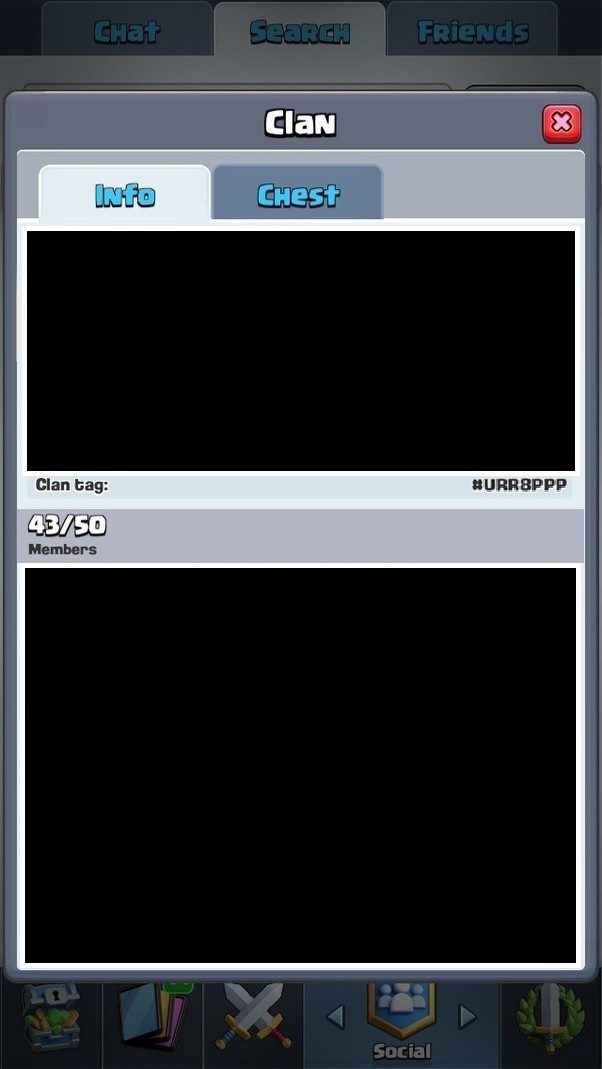
Clash Royale CLAN TAG#URR8PPP
How to correct the output of this jquery code?
This code should print:
But it always shows 2 or 3 randomly when the 4th condition is true.
whats the problem in here? Take a look at the console.
https://codepen.io/code_alex/pen/xJLwpq
var screenWidth = $(window).width();
if(screenWidth < 740 || screenWidth == 740) {
console.log("1");
} else if(screenWidth > 740 && screenWidth < 1065){
console.log("2");
} else if(screenWidth > 1065 || screenWidth == 1065) {
console.log("3");
} else if (screenWidth > 1389 || screenWidth == 1389) {
console.log("4");
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<=
>=
$(window).width()
screen.width
console.log(screenWidth)
if
jQuery
@media
3 Answers
3
The conditions set correctly would look like below.
Consider @media rules unless you really need that input in JS / jQuery.
var screenWidth = $(window).width();
console.log(screenWidth);
if(screenWidth <= 740) {
console.log("1");
} else if(screenWidth > 740 && screenWidth <= 1065){
console.log("2");
} else if(screenWidth > 1065 && screenWidth <= 1389) {
console.log("3");
} else if (screenWidth > 1389) {
console.log("4");
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
Additionnaly, you should look for common breakpoints.
Once one branch of an else if
is true then the execution will end, rather than checking to see if another is also true. By your logic, if you have a screenWidth
of 1400
then screenWidth > 1065 || screenWidth == 1065
is true, so you don't reach the screenWidth > 1389 || screenWidth == 1389
check.
else if
screenWidth
1400
screenWidth > 1065 || screenWidth == 1065
screenWidth > 1389 || screenWidth == 1389
You could revise your code to:
var screenWidth = $(window).width();
if (screenWidth <= 740) {
console.log("1");
} else if(screenWidth > 740 && screenWidth < 1065){
console.log("2");
} else if(screenWidth >= 1065 && screenWidth < 1389) {
console.log("3");
} else if (screenWidth >= 1389) {
console.log("4");
}
Or you can do
var i,warr=[0,740,1065,1389],
w=screen.width;
for (i=0;warr[i]<=w;i++){}
console.log(i);
@Barmar: thanks for the hint re: screen.width
!
screen.width
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Side note:
<=
is less than or equal.>=
is greater than or equal.– Taplar
yesterday