Tuple conversion in python to list
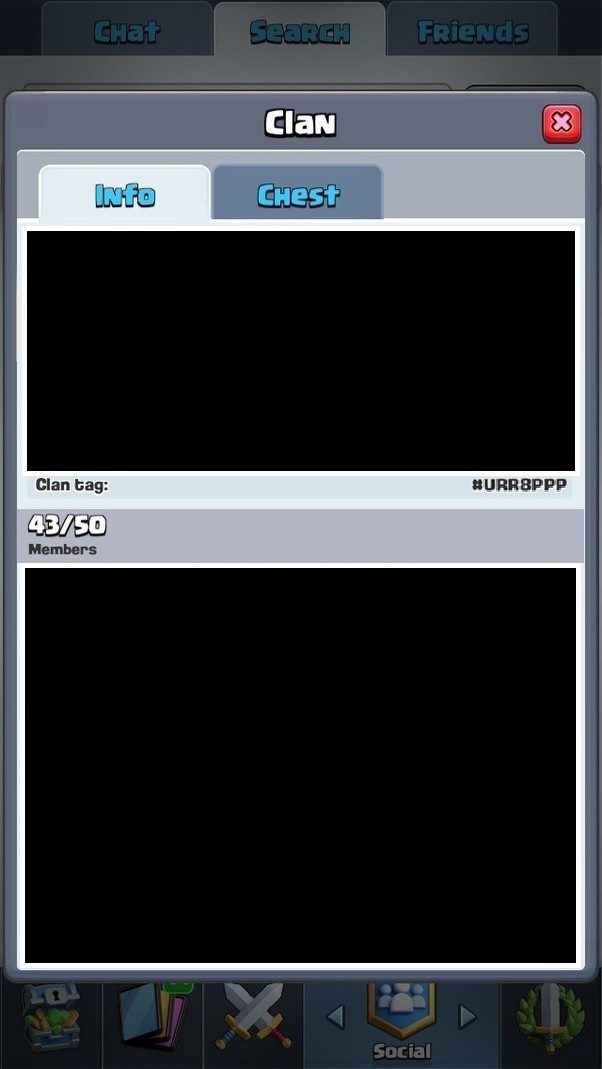

Tuple conversion in python to list
I have a tuple like
a = (1,2,3,4).
Is it possible to change the tuple to
a = [('roll', 1),('roll',2),('roll', 3),('roll', 4)]
a
a
5 Answers
5
Simple list comprehension here -
a = [("roll", i) for i in a]
OP
[('roll', 1), ('roll', 2), ('roll', 3), ('roll', 4)]
More about list comprehension here
@nikolas your welcome, do see the edited answer
– ThatBird
yesterday
Yes - simply do a list comprehension:
a = [('roll', i) for i in a]
Note that doing this will rebind a
! (See the note by @ShadowRanger.)
a
Technically, it rebinds
a
; if a
is aliased elsewhere under a different name, that alias is unchanged (e.g. b = a; a = [('roll', i) for i in a]
will preserve the original value of a
, accessible through the b
alias). Subtle distinction, but important in some cases. Since a
is initially bound to a tuple
, it's not possible to mutate the tuple
itself.– ShadowRanger
yesterday
a
a
b = a; a = [('roll', i) for i in a]
a
b
a
tuple
tuple
Thanks, @ShadowRanger! That's very informative - I've edited my answer to reflect that. Thanks again!
– jrd1
yesterday
Easily. With a list comprehension:
a = [("roll", x) for x in a]
or with itertools
stuff:
itertools
import itertools
a = list(zip(itertools.repeat('roll'), a)) # No need to wrap in list if you'll iterate the result and discard it
You can also use map
function:
map
i = (1,2,3,4)
list(map(lambda x: ('roll',x), i))
Note: Any use of
map
that requires a lambda
to work is more efficiently and more clearly expressed as a list comprehension or generator expression, since the listcomp/genexpr can inline the work done in the lambda
, avoiding (relatively expensive) function call overhead for trivial work.– ShadowRanger
yesterday
map
lambda
lambda
Or another alternative using list()
list(zip(["roll"]*4, a))
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
One does not simply "change the tuple." You can change the value of the name
a
to be the appropriate tuple, but tuples are immutable and cannot themselves be changed. In other words, you changea
, not the tuple.– Rory Daulton
yesterday