![Creative The name of the picture]()
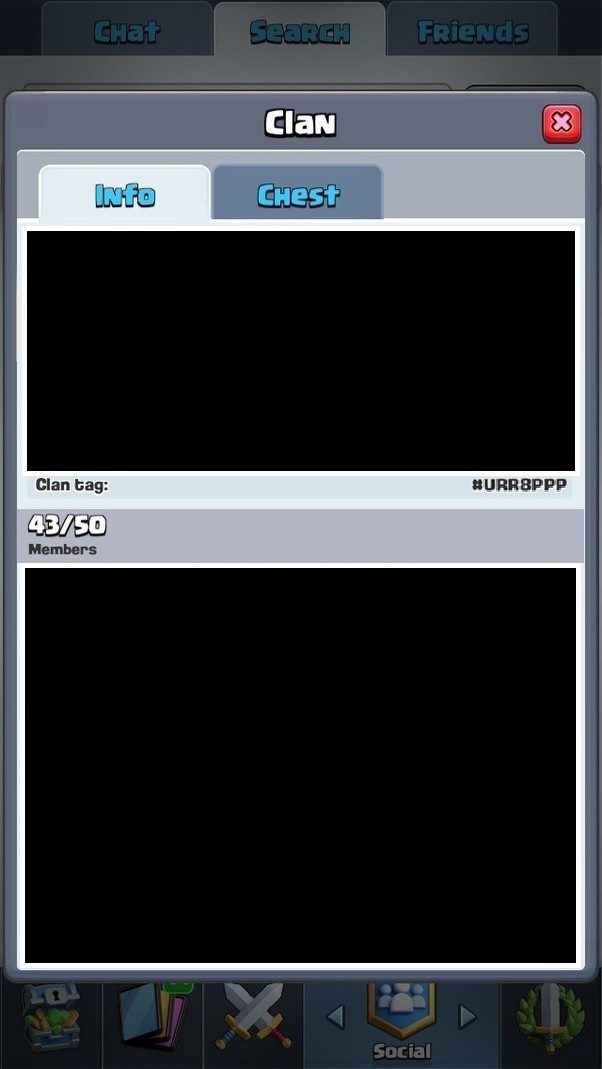
Clash Royale CLAN TAG#URR8PPP
In C#, what is a good method for dealing with long template type names that include protected nested types?
I have a C# class with methods that take and return types that look something like this:
Dictionary<ClassWithLongNameA, Dictionary<ClassWithLongNameB, ClassWithLongNameB.ProtectedStructType> >
or this:
Dictionary<ClassWithLongNameB, ClassWithLongNameB.ProtectedStructType>
That makes for some cumbersome method definitions that are long and hard to read. If this were C++ I would make my code more readable by utilizing a typedef but that's not available in C#.
I'm aware that I could use a using
statement in a manner similar to typedef
in C++ from reading this question, but it doesn't work for me for two reasons:
using
typedef
It only applies to one source file and I need this to work with all of the source files that utilize my class and/or inherit from it
I can't use it if one of the template arguments is a protected or private nested type (as it won't be accessible to the using
statement) and that is the case for me
using
I didn't see any other answers in that question that seemed like a good solution to my issue specifically.
What are some other good ways of dealing with this?
edit: This question is not a duplicate of this question as every answer in that thread suggests a using
statement, and I have already explained why that is not an appropriate answer to my question.
using
using
public class Alias : ReallyLongType<A, B>
2 Answers
2
As suggested in my comment, you could create an alias class that derives from the very complex type name, which would get around your issue of using
aliases only applying to a single source file.
using
public class LongTypeAlias : Dictionary<ClassWithLongNameA, Dictionary<ClassWithLongNameB, ClassWithLongNameB.ProtectedStructType>>
{
}
I'll try and cover some of the benefits/drawbacks, but these lists are by no means comprehensive.
Benefits:
using
var
Drawbacks:
System.Type
System.Type
System.Type
Of course, none of this covers the root question of why the types are so long in the first place. I would encourage you to attempt refactoring where you are able - ReSharper (and even VS2017 I think) provide a lot of excellent tools that make renaming, extracting classes, etc. very easy.
Subclass the Dictionary with a shorter name. You don't even need a body.
class ShortName : Dictionary<ClassWithLongNameB, ClassWithLongNameB.ProtectedStructType> {}
Then you can declare your methods with that class.
public ShortName GetDictionary()
{
var dict = new ShortName();
dict.Add
(
new ClassWithLongNameB(),
new ClassWithLongNameB.ProtectedStructType()
};
return dict;
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Possible duplicate of C#: How to create "aliases" for classes
– Kacper
yesterday