Converting Python Dictionary to JSON Array
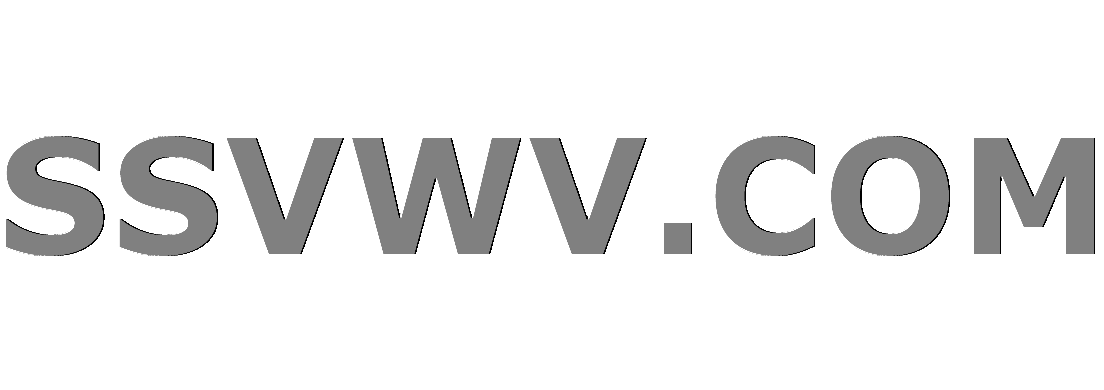
Multi tool use
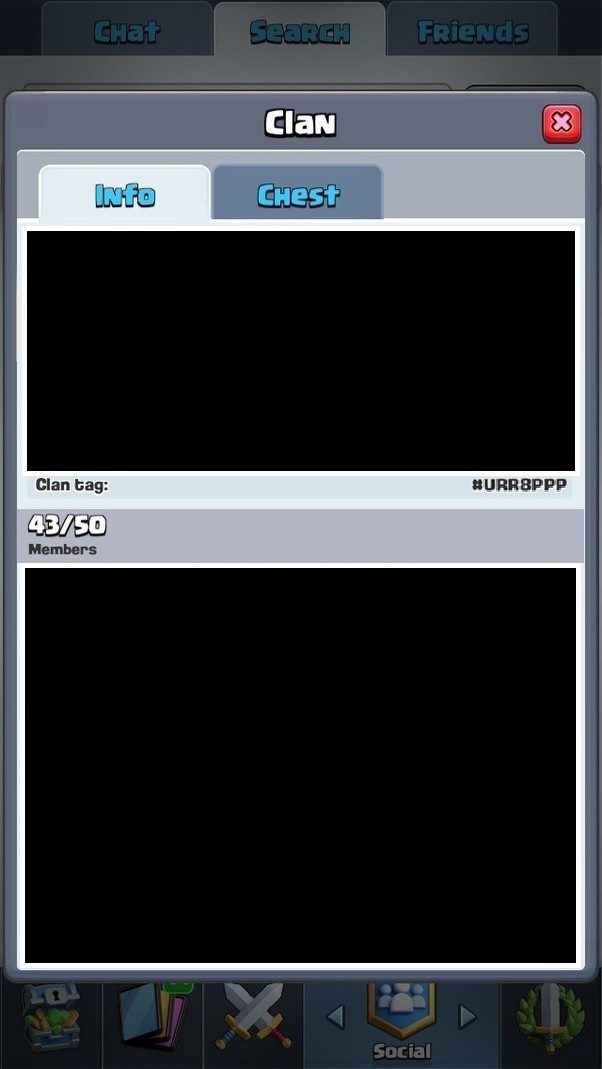

Converting Python Dictionary to JSON Array
I currently have a Python Dictionary that looks something like this:
OrderedDict([('2017-07-24', 149.7619), ('2017-07-25', 150.4019), ('2017-07-26', 151.1109), ...
that I am converting to JSON like so:
one_yr = json.dumps(priceDict)
Currently I am adding values to the dictionary from an SQL query by looping through it like so:
for i in query:
date = i[0]
close = i[1]
priceDict[date] = close
The problem is that this returns a JSON object, that i then have to convert to a JSON array.
I am wondering if I can just convert my Python Dictionary to a JSON array directly? Thanks.
3 Answers
3
json.dumps(list(priceDict.items()))
But why do you have an OrderedDict
in first place? If you pass the same list you passed to OrderedDict
to json.dumps
it will generate your array:
OrderedDict
OrderedDict
json.dumps
json.dumps([('2017-07-24', 149.7619), ('2017-07-25', 150.4019),....])
No need for OrderedDict
in this case
OrderedDict
TypeError: Object of type odict_items is not JSON serializable
? json.dumps(list(price_dict.items()))
?– G_M
yesterday
TypeError: Object of type odict_items is not JSON serializable
json.dumps(list(price_dict.items()))
Thanks for the response, I should have mentioned I am using a Highcharts (a javascript charting library), that depends on the data being in an array. Currently the data looks like so:
var one_yr = [["2017-07-24", 41.8175], ["2017-07-25", 41.9946], ... ["2018-01-01", 42.6143]}
and isn't being plotted.– ng150716
yesterday
var one_yr = [["2017-07-24", 41.8175], ["2017-07-25", 41.9946], ... ["2018-01-01", 42.6143]}
I removed the OrderedDict but kept all the other data, I think I understand the request. See if this works for you:
import json
my_dict = ([('2017-07-24', 149.7619), ('2017-07-25', 150.4019), ('2017-07-26', 151.1109)])
print(json.dumps(my_dict, indent=4, sort_keys=True))
were you able to get this to work?
– Steven M
yesterday
Unfortunately no, this still seems to create an object not an array (the data is still encapuslated in curly braces
{ ... }
as opposed to brackets [ ... ]
– ng150716
yesterday
{ ... }
[ ... ]
If you want to convert a Python Dictionary to JSON using the json.dumps() method.
`
import json
from decimal import Decimal
d = {}
d["date"] = "2017-07-24"
d["quantity"] = "149.7619"
print json.dumps(d, ensure_ascii=False)
`
Unfortunately this seems to create a dict with only two values. IE:
[('close', 149.7619), ('date', '2017-07-24')]
, the problem is I am looping through a series of values.– ng150716
yesterday
[('close', 149.7619), ('date', '2017-07-24')]
@ng150716 you can keep on adding the values by
d["close"] = "149.7619,150.4019"
– Vibhor Karnawat
yesterday
d["close"] = "149.7619,150.4019"
Sorry, I'm very new to serializing data and a bit rusty with Python over-all, how would that fit into for loop?
– ng150716
yesterday
@ng150716their is no need of loop I guess
d[""] = ([('2017-07-24', 149.7619), ('2017-07-25', 150.4019), ('2017-07-26', 151.1109,...)])
this might help you– Vibhor Karnawat
yesterday
d[""] = ([('2017-07-24', 149.7619), ('2017-07-25', 150.4019), ('2017-07-26', 151.1109,...)])
Sorry, i don't think I explained clearly what i mean't, but I have to loop through an SQL query to add the values to the dict. I have updated my post to show what I mean.
– ng150716
yesterday
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
But this is a dict. A dict maps to an object. If you don't want an object, why are you passing a dict?
– Daniel Roseman
yesterday