![Creative The name of the picture]()
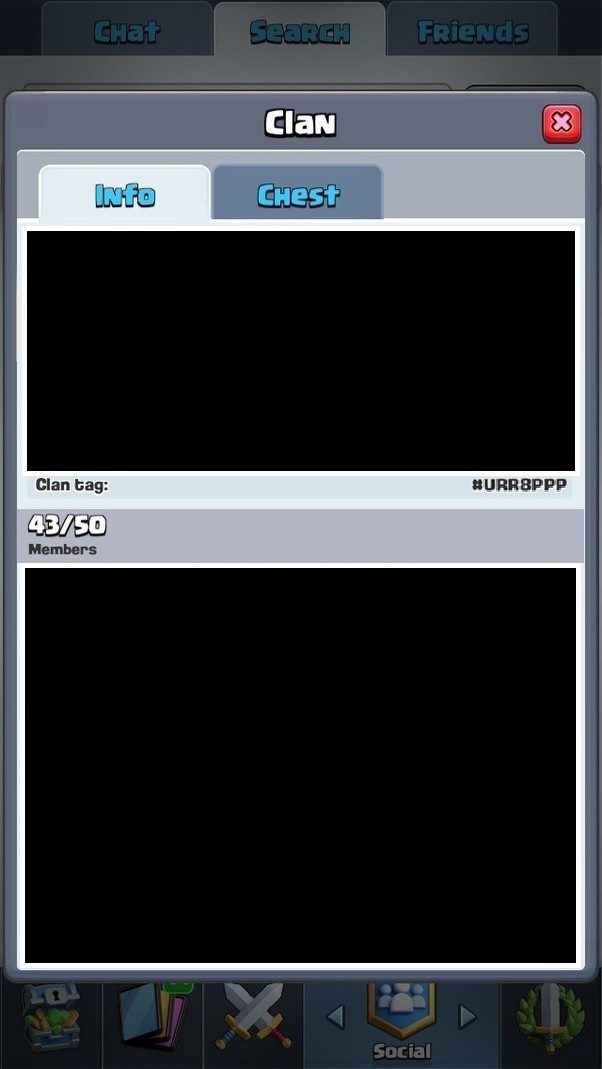
Clash Royale CLAN TAG#URR8PPP
How to get a random color for DeckGL PolygonLayers?
Kindly can someone help me create a code, that allows me to have a different color for each trips?
render() {
const {viewport, buildings, trips, trailLength, time} = this.props;
if (!buildings || !trips) {
return null;
}
const layers = [
new TripsLayer({
id: 'trips',
data: trips,
getPath: d => d.segments,
getColor: d => (d.vendor === 0 ? [0, 255, 0] : [0, 255, 0]),
opacity: 0.3,
strokeWidth: 2,
trailLength,
currentTime: time
}),
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: f => [0, 255, 0],
lightSettings: LIGHT_SETTINGS
})
];
return <DeckGL {...viewport} layers={layers} />;
}
Original Screenshot
I'm using the libraries in the deck.gl-5.3-release repository.
Thank you very much, I am at the beginning and I do not know how to do it.
getFillColor
PolygonLayer
7 Answers
7
HSV (Hue, Saturation, Value) depicts the colors as a color circle, which you can just rotate around, so you can just increment an integer and use the HSV => RGB conversion. If you want more information on HSV and the conversion to RGB you can find it here on Wikipedia.
This could lead you to a function as this:
const rainbow = (function () {
let
hue = 0,
increment = 10;
return function () {
// HSV => RGB conversion, for details see
// https://en.wikipedia.org/wiki/HSL_and_HSV#From_HSV
let
h = Math.floor(hue / 60),
v = 1, // lock value (brightness) at 100%
f = hue / 60 - h,
p = 0,
q = 1 - f,
t = 0,
r, g, b;
// assign RGB values based on HSV color circle segment
// RGB values are between 0 and 1
if (h === 0) [r, g, b] = [v, t, p];
if (h === 1) [r, g, b] = [q, v, p];
if (h === 2) [r, g, b] = [p, v, t];
if (h === 3) [r, g, b] = [p, q, v];
if (h === 4) [r, g, b] = [t, p, v];
if (h === 5) [r, g, b] = [v, p, q];
// transform to range [0, 255]
[r, g, b] = [r, g, b].map(v => Math.ceil(v * 255));
// increment hue index
hue += increment;
// and keep it between 0-360
hue %= 360;
// return RGB array
return [r, g, b];
};
})();
Note:
I have implemented this in a way that locks the saturation and value at 100%.
Every time you call rainbow()
you get the next color increment.
rainbow()
To change how much the next color differs from the previous, you can set increment
to a higher or lower value.
increment
getFillColor
accepts either a Function or an Array.
If you give it an RGB Array, all polygons will use that color.
But if you give it a function, the function will be called for each polygon.
getFillColor
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: rainbow(),
lightSettings: LIGHT_SETTINGS
})
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: rainbow,
lightSettings: LIGHT_SETTINGS
})
You can find more information about deck.gl PolygonLayer getFillColor
on their GitHub page
getFillColor
But this is pretty much coding for you, so I hope you can see where you went wrong and learn from it :)
import React, {
Component
} from 'react';
import DeckGL, {
PolygonLayer
} from 'deck.gl';
import TripsLayer from './trips-layer';
const LIGHT_SETTINGS = {
lightsPosition: [-74.05, 40.7, 8000, -73.5, 41, 5000],
ambientRatio: 0.05,
diffuseRatio: 0.6,
specularRatio: 0.8,
lightsStrength: [2.0, 0.0, 0.0, 0.0],
numberOfLights: 2
};
const rainbow = (function() {
let
hue = 0,
increment = 10;
return function() {
// HSV => RGB conversion, for details see
// https://en.wikipedia.org/wiki/HSL_and_HSV#From_HSV
let
h = Math.floor(hue / 60),
v = 1, // lock value (brightness) at 100%
f = hue / 60 - h,
p = 0,
q = 1 - f,
t = 0,
r, g, b;
// assign RGB values based on HSV color circle segment
// RGB values are between 0 and 1
if (h === 0)[r, g, b] = [v, t, p];
if (h === 1)[r, g, b] = [q, v, p];
if (h === 2)[r, g, b] = [p, v, t];
if (h === 3)[r, g, b] = [p, q, v];
if (h === 4)[r, g, b] = [t, p, v];
if (h === 5)[r, g, b] = [v, p, q];
// transform to range [0, 255]
[r, g, b] = [r, g, b].map(v => Math.ceil(v * 255));
// increment hue index
hue += increment;
// and keep it between 0-360
hue %= 360;
// return RGB array
return [r, g, b];
};
})();
export default class DeckGLOverlay extends Component {
static get defaultViewport() {
return {
longitude: 9.196471,
latitude: 45.457692,
zoom: 15,
maxZoom: 20,
pitch: 45,
bearing: 0
};
}
render() {
const {viewport, buildings, trips, trailLength, time} = this.props;
if (!buildings || !trips) {
return null;
}
const layers = [
new TripsLayer({
id: 'trips',
data: trips,
getPath: d => d.segments,
getColor: d => (d.vendor === 0 ? [0, 255, 0] : [0, 255, 0]),
opacity: 0.3,
strokeWidth: 2,
trailLength,
currentTime: time
}),
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: rainbow,
lightSettings: LIGHT_SETTINGS
})
];
return <DeckGL { ...viewport } layers = { layers } />;
}
}
In the line getFillColor: this.rainbow,
, this will make every Polygon a different color. If you want a constant color for a PolygonLayer, you have to replace it with getFillColor: this.rainbow(),
(added brackets).
getFillColor: this.rainbow,
getFillColor: this.rainbow(),
import React, {Component} from 'react';
import DeckGL, {PolygonLayer} from 'deck.gl';
import TripsLayer from './trips-layer';
const LIGHT_SETTINGS = {
lightsPosition: [-74.05, 40.7, 8000, -73.5, 41, 5000],
ambientRatio: 0.05,
diffuseRatio: 0.6,
specularRatio: 0.8,
lightsStrength: [2.0, 0.0, 0.0, 0.0],
numberOfLights: 2
};
export default class DeckGLOverlay extends Component {
static get defaultViewport() {
return {
longitude: 9.196471,
latitude: 45.457692,
zoom: 15,
maxZoom: 20,
pitch: 45,
bearing: 0
};
}
render() {
const rainbow = (function () {
let
hue = 0,
increment = 10;
return function () {
// HSV => RGB conversion, for details see
// https://en.wikipedia.org/wiki/HSL_and_HSV#From_HSV
let
h = Math.floor(hue / 60),
v = 1, // lock value (brightness) at 100%
f = hue / 60 - h,
p = 0,
q = 1 - f,
t = 0,
r, g, b;
// assign RGB values based on HSV color circle segment
// RGB values are between 0 and 1
if (h === 0) [r, g, b] = [v, t, p];
if (h === 1) [r, g, b] = [q, v, p];
if (h === 2) [r, g, b] = [p, v, t];
if (h === 3) [r, g, b] = [p, q, v];
if (h === 4) [r, g, b] = [t, p, v];
if (h === 5) [r, g, b] = [v, p, q];
// transform to range [0, 255]
[r, g, b] = [r, g, b].map(v => Math.ceil(v * 255));
// increment hue index
hue += increment;
// and keep it between 0-360
hue %= 360;
// return RGB array
return [r, g, b];
};
})();
}
const layers = [
new TripsLayer({
id: 'trips',
data: trips,
getPath: d => d.segments,
getColor: d => (d.vendor === 0 ? [0, 255, 0] : [0, 255, 0]),
opacity: 0.3,
strokeWidth: 2,
trailLength,
currentTime: time
}),
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: rainbow,
lightSettings: LIGHT_SETTINGS
})
];
return <DeckGL {...viewport} layers={layers} />;
}
}
Time: 4370ms
Built at: 2018-07-27 10:43:48
Asset Size Chunks Chunk Names
app.js 2.46 MiB app [emitted] app
Entrypoint app = app.js
[./app.js] 4.79 KiB {app} [built]
[./deckgl-overlay.js] 398 bytes {app} [built] [failed] [1 error]
[./node_modules/loglevel/lib/loglevel.js] 7.68 KiB {app} [built]
[./node_modules/react-dom/index.js] 1.33 KiB {app} [built]
[./node_modules/react-map-gl/dist/esm/index.js] 903 bytes {app} [built]
[./node_modules/url/url.js] 22.8 KiB {app} [built]
[./node_modules/webpack-dev-server/client/index.js?http://localhost:8080] (webpack)-dev-server/client?http://localhost:8080 7.75 KiB {app} [built]
[./node_modules/webpack-dev-server/client/socket.js] (webpack)-dev-server/client/socket.js 1.05 KiB {app} [built]
[0] multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./app.js 52 bytes {app} [built]
[./node_modules/webpack-dev-server/node_modules/strip-ansi/index.js] (webpack)-dev-server/node_modules/strip-ansi/index.js 161 bytes {app} [built]
[./node_modules/webpack/hot sync ^./log$] (webpack)/hot sync nonrecursive ^./log$ 170 bytes {app} [built]
[./node_modules/webpack/hot/dev-server.js] (webpack)/hot/dev-server.js 1.61 KiB {app} [built]
[./node_modules/webpack/hot/emitter.js] (webpack)/hot/emitter.js 75 bytes {app} [built]
[./node_modules/webpack/hot/log-apply-result.js] (webpack)/hot/log-apply-result.js 1.27 KiB {app} [built]
[./node_modules/webpack/hot/log.js] (webpack)/hot/log.js 1010 bytes {app} [built]
+ 179 hidden modules
WARNING in EnvironmentPlugin - MapboxAccessToken environment variable is undefined.
You can pass an object with default values to suppress this warning.
See https://webpack.js.org/plugins/environment-plugin for example.
ERROR in ./deckgl-overlay.js
Module build failed (from ./node_modules/buble-loader/index.js):
BubleLoaderError:
61 : };
62 : })();
63 : }
64 :
65 : const layers = [
^
Unexpected token (65:10)
at Object.module.exports (C:UserselombardoDesktopuberpiemonte_3deck.gl-5.3-releaseexampleswebsitetripsnode_modulesbuble-loaderindex.js:24:69)
@ ./app.js 5:0-48 73:34-47 154:29-42
@ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./app.js
i 「wdm」: Failed to compile.
import React, {
Component
} from 'react';
import DeckGL, {
PolygonLayer
} from 'deck.gl';
import TripsLayer from './trips-layer';
const LIGHT_SETTINGS = {
lightsPosition: [-74.05, 40.7, 8000, -73.5, 41, 5000],
ambientRatio: 0.05,
diffuseRatio: 0.6,
specularRatio: 0.8,
lightsStrength: [2.0, 0.0, 0.0, 0.0],
numberOfLights: 2
};
export default class DeckGLOverlay extends Component {
static get defaultViewport() {
return {
longitude: 9.196471,
latitude: 45.457692,
zoom: 15,
maxZoom: 20,
pitch: 45,
bearing: 0
};
}
rainbow = (function () {
let
hue = 0,
increment = 10;
return function () {
// HSV => RGB conversion, for details see
// https://en.wikipedia.org/wiki/HSL_and_HSV#From_HSV
let
h = Math.floor(hue / 60),
v = 1, // lock value (brightness) at 100%
f = hue / 60 - h,
p = 0,
q = 1 - f,
t = 0,
r, g, b;
// assign RGB values based on HSV color circle segment
// RGB values are between 0 and 1
if (h === 0)[r, g, b] = [v, t, p];
if (h === 1)[r, g, b] = [q, v, p];
if (h === 2)[r, g, b] = [p, v, t];
if (h === 3)[r, g, b] = [p, q, v];
if (h === 4)[r, g, b] = [t, p, v];
if (h === 5)[r, g, b] = [v, p, q];
// transform to range [0, 255]
[r, g, b] = [r, g, b].map(v => Math.ceil(v * 255));
// increment hue index
hue += increment;
// and keep it between 0-360
hue %= 360;
// return RGB array
return [r, g, b];
};
})()
render() {
const layers = [
new TripsLayer({
id: 'trips',
data: trips,
getPath: d => d.segments,
getColor: d => (d.vendor === 0 ? [0, 255, 0] : [0, 255, 0]),
opacity: 0.3,
strokeWidth: 2,
trailLength,
currentTime: time
}),
new PolygonLayer({
id: 'buildings',
data: buildings,
extruded: true,
wireframe: false,
fp64: true,
opacity: 0.5,
getPolygon: f => f.polygon,
getElevation: f => f.height,
getFillColor: this.rainbow,
lightSettings: LIGHT_SETTINGS
})
];
return <DeckGL { ...viewport } layers = { layers } />;
}
}

Failed to load resource: the server responded with a status of 404 (Not Found)
client:71 [WDS] Hot Module Replacement enabled.
client:155 [WDS] Errors while compiling. Reload prevented.
errors @ client:155
client:161 ./deckgl-overlay.js
Module build failed (from ./node_modules/buble-loader/index.js):
BubleLoaderError:
26 : bearing: 0
27 : };
28 : }
29 :
30 : rainbow = (function () {
^
Unexpected token (30:12)
at Object.module.exports (C:UserselombardoDesktopubercolordeck.gl-5.3-releaseexampleswebsitetripsnode_modulesbuble-loaderindex.js:24:69)
errors @ client:161
Uncaught ReferenceError: trips is not defined
at DeckGLOverlay.render (deckgl-overlay.js:85)
at finishClassComponent (react-dom.development.js:13193)
at updateClassComponent (react-dom.development.js:13155)
at beginWork (react-dom.development.js:13824)
at performUnitOfWork (react-dom.development.js:15863)
at workLoop (react-dom.development.js:15902)
at HTMLUnknownElement.callCallback (react-dom.development.js:100)
at Object.invokeGuardedCallbackDev (react-dom.development.js:138)
at invokeGuardedCallback (react-dom.development.js:187)
at replayUnitOfWork (react-dom.development.js:15310)
react-dom.development.js:14226 The above error occurred in the <DeckGLOverlay> component:
in DeckGLOverlay (created by Root)
in div (created by StaticMap)
in div (created by StaticMap)
in StaticMap (created by InteractiveMap)
in div (created by InteractiveMap)
in InteractiveMap (created by Root)
in Root
Consider adding an error boundary to your tree to customize error handling behavior.
logCapturedError @ react-dom.development.js:14226
react-dom.development.js:16543 Uncaught ReferenceError: trips is not defined
at DeckGLOverlay.render (deckgl-overlay.js:85)
at finishClassComponent (react-dom.development.js:13193)
at updateClassComponent (react-dom.development.js:13155)
at beginWork (react-dom.development.js:13824)
at performUnitOfWork (react-dom.development.js:15863)
at workLoop (react-dom.development.js:15902)
at renderRoot (react-dom.development.js:15942)
at performWorkOnRoot (react-dom.development.js:16560)
at performWork (react-dom.development.js:16482)
at performSyncWork (react-dom.development.js:16454)
[![ok it works !! but the output that comes out of me is not the desired one. I'll explain how you can see in the new image that I entered all the lines that represent a journey are of the same color. Instead, I would like every trip to have a different color][ok it works !! but the output that comes out of me is not the desired one. I'll explain how you can see in the new image that I entered all the lines that represent a journey are of the same color. Instead, I would like every trip to have a different color]][ok it works !! but the output that comes out of me is not the desired one. I'll explain how you can see in the new image that I entered all the lines that represent a journey are of the same color. Instead, I would like every trip to have a different color]
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Welcome to SO. Please take a tour of the help centre to see how to ask a good question. We cannot help you if you do not provide any code - see how to create a Minimal, Complete, and Verifiable example (pictures of code do not count as code - please eit your question using the snippet button to add actual code)
– Pete
yesterday