![Creative The name of the picture]()
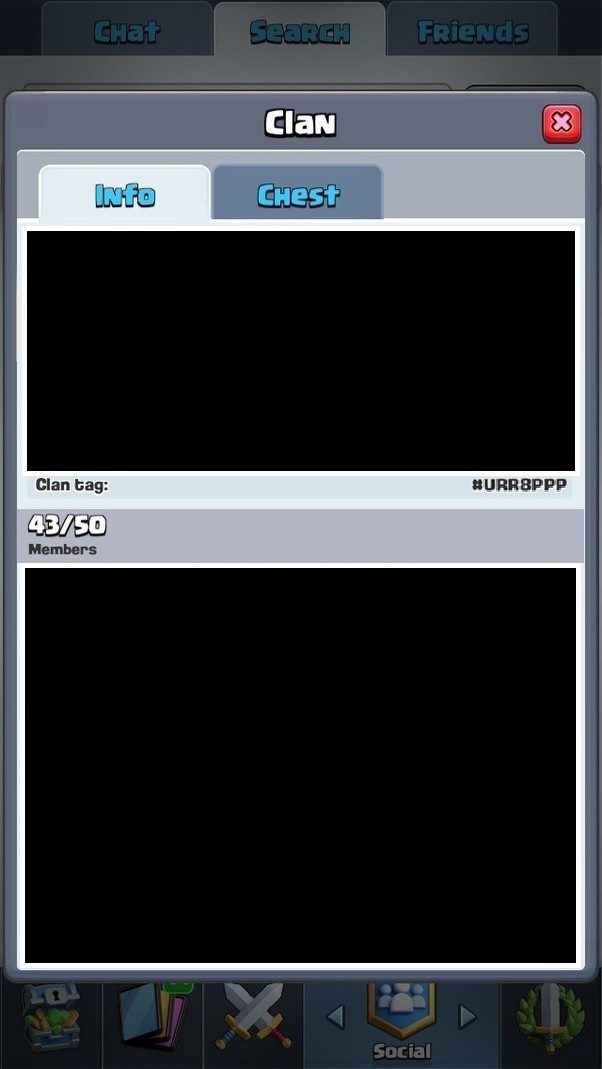
Clash Royale CLAN TAG#URR8PPP
How to read AppSettings values from Config.json in ASP.NET Core
I have got my AppSettings setup in Config.json like this:
{
"AppSettings": {
"token": "1234"
}
}
I have searched online on how to read AppSettings values from config.json but I could not get anything useful.
I tried:
var configuration = new Configuration();
var appSettings = configuration.Get("AppSettings"); // null
var token = configuration.Get("token"); // null
I know with ASP.NET 4.0 you can do this:
System.Configuration.ConfigurationManager.AppSettings["token"];
But how do I do this in ASP.NET Core?
9 Answers
9
This has had a few twists and turns. I've modified this answer to be up to date with ASP.NET Core 2.0 (as of 26/02/2018).
This is mostly taken from the official documentation:
To work with settings in your ASP.NET application, it is recommended that you only instantiate a Configuration
in your application’s Startup
class. Then, use the Options pattern to access individual settings. Let's say we have an appsettings.json
file that looks like this:
Configuration
Startup
appsettings.json
{
"ApplicationName": "MyApp",
"Version": "1.0.0"
}
And we have a POCO object representing the configuration:
public class MyConfig
{
public string ApplicationName { get; set; }
public int Version { get; set; }
}
Now we build the configuration in Startup.cs
:
Startup.cs
public class Startup
{
public IConfigurationRoot Configuration { get; set; }
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true);
Configuration = builder.Build();
}
}
Note that appsettings.json
will be registered by default in .NET Core 2.0. We can also register an appsettings.{Environment}.json
config file per environment if needed.
appsettings.json
appsettings.{Environment}.json
If we want to inject our configuration to our controllers, we'll need to register it with the runtime. We do so via Startup.ConfigureServices
:
Startup.ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
// Add functionality to inject IOptions<T>
services.AddOptions();
// Add our Config object so it can be injected
services.Configure<MyConfig>(Configuration.GetSection("MyConfig"));
}
And we inject it like this:
public class HomeController : Controller
{
private readonly IOptions<MyConfig> config;
public HomeController(IOptions<MyConfig> config)
{
this.config = config;
}
// GET: /<controller>/
public IActionResult Index() => View(config.Value);
}
The full Startup
class:
Startup
public class Startup
{
public IConfigurationRoot Configuration { get; set; }
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true);
Configuration = builder.Build();
}
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
// Add functionality to inject IOptions<T>
services.AddOptions();
// Add our Config object so it can be injected
services.Configure<MyConfig>(Configuration.GetSection("MyConfig"));
}
}
Configuration does not contain a definition for 'AddJsonFile'...
"1.0.0-beta4"
"1.0.0-alpha4"
Microsoft.Extensions.Options.ConfigurationExtensions
First off:
The assembly name and namespace of Microsoft.Framework.ConfigurationModel has changed to Microsoft.Framework.Configuration. So you should use:
e.g.
"Microsoft.Framework.Configuration.Json": "1.0.0-beta7"
as a dependency in project.json
. Use beta5 or 6 if you don't have 7 installed.
Then you can do something like this in Startup.cs
.
project.json
Startup.cs
public IConfiguration Configuration { get; set; }
public Startup(IHostingEnvironment env, IApplicationEnvironment appEnv)
{
var configurationBuilder = new ConfigurationBuilder(appEnv.ApplicationBasePath)
.AddJsonFile("config.json")
.AddEnvironmentVariables();
Configuration = configurationBuilder.Build();
}
If you then want to retrieve a variable from the config.json you can get it right away using:
public void Configure(IApplicationBuilder app)
{
// Add .Value to get the token string
var token = Configuration.GetSection("AppSettings:token");
app.Run(async (context) =>
{
await context.Response.WriteAsync("This is a token with key (" + token.Key + ") " + token.Value);
});
}
or you can create a class called AppSettings like this:
public class AppSettings
{
public string token { get; set; }
}
and configure the services like this:
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.Configure<MvcOptions>(options =>
{
//mvc options
});
services.Configure<AppSettings>(Configuration.GetSection("AppSettings"));
}
and then access it through e.g. a controller like this:
public class HomeController : Controller
{
private string _token;
public HomeController(IOptions<AppSettings> settings)
{
_token = settings.Options.token;
}
}
For .NET Core 2.0, things have changed a little bit. The startup constructor takes a Configuration object as a parameter, So using the ConfigurationBuilder
is not required. Here is mine:
ConfigurationBuilder
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.Configure<StorageOptions>(Configuration.GetSection("AzureStorageConfig"));
}
My POCO is the StorageOptions
object mentioned at the top:
StorageOptions
namespace Brazzers.Models
{
public class StorageOptions
{
public String StorageConnectionString { get; set; }
public String AccountName { get; set; }
public String AccountKey { get; set; }
public String DefaultEndpointsProtocol { get; set; }
public String EndpointSuffix { get; set; }
public StorageOptions() { }
}
}
And the configuration is actually a subsection of my appsettings.json
file, named AzureStorageConfig
:
appsettings.json
AzureStorageConfig
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\mssqllocaldb;",
"StorageConnectionString": "DefaultEndpointsProtocol=https;AccountName=brazzerswebapp;AccountKey=Cng4Afwlk242-23=-_d2ksa69*2xM0jLUUxoAw==;EndpointSuffix=core.windows.net"
},
"Logging": {
"IncludeScopes": false,
"LogLevel": {
"Default": "Warning"
}
},
"AzureStorageConfig": {
"AccountName": "brazzerswebapp",
"AccountKey": "Cng4Afwlk242-23=-_d2ksa69*2xM0jLUUxoAw==",
"DefaultEndpointsProtocol": "https",
"EndpointSuffix": "core.windows.net",
"StorageConnectionString": "DefaultEndpointsProtocol=https;AccountName=brazzerswebapp;AccountKey=Cng4Afwlk242-23=-_d2ksa69*2xM0jLUUxoAw==;EndpointSuffix=core.windows.net"
}
}
The only thing I'll add is that, since the constructor has changed, I haven't tested whether something extra needs to be done for it to load appsettings.<environmentname>.json
as opposed to appsettings.json
.
appsettings.<environmentname>.json
appsettings.json
public HomeController(IOptions<StorageOptions> settings)
If you just want to get the value of the token then use
Configuration["AppSettings:token"]
Configuration["AppSettings:token"]
They just keep changing things – having just updated VS and had the whole project bomb, on the road to recovery and the new way looks like this:
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true);
if (env.IsDevelopment())
{
// For more details on using the user secret store see http://go.microsoft.com/fwlink/?LinkID=532709
builder.AddUserSecrets();
}
builder.AddEnvironmentVariables();
Configuration = builder.Build();
}
I kept missing this line!
.SetBasePath(env.ContentRootPath)
So I doubt this is good practice but it's working locally, I'll update this if it fails when I publish/deploy (to an IIS web service).
Step 1.) Add this assembly to the top of your class (in my case, controller class):
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Configuration;
Step 2.) Add this or something like it:
var config = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json").Build();
var config = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json").Build();
Step 3.) Call your key's value by doing this (returns string):
config["NameOfYourKey"]
config["NameOfYourKey"]
Just to complement the Yuval Itzchakov answer.
You can load configuration without builder function, you can just inject it.
public IConfiguration Configuration { get; set; }
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
For .NET Core 2.0, you can simply:
Declare your key/value pairs in appsettings.json:
{
"MyKey": "MyValue
}
Inject the configuration service in startup.cs, and get the value using the service
using Microsoft.Extensions.Configuration;
public class Startup
{
public void Configure(IConfiguration configuration,
... other injected services
)
{
app.Run(async (context) =>
{
string myValue = configuration["MyKey"];
await context.Response.WriteAsync(myValue);
});
Was this "cheating"? I just made my Configuration in the Startup class static, and then I can access it from anywhere else:
public class Startup
{
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true)
.AddEnvironmentVariables();
Configuration = builder.Build();
}
public static IConfiguration Configuration { get; set; }
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
possible duplicate of ASP.NET 5 (vNext) - Getting a Configuration Setting
– mason
Jul 16 '15 at 13:20