![Creative The name of the picture]()
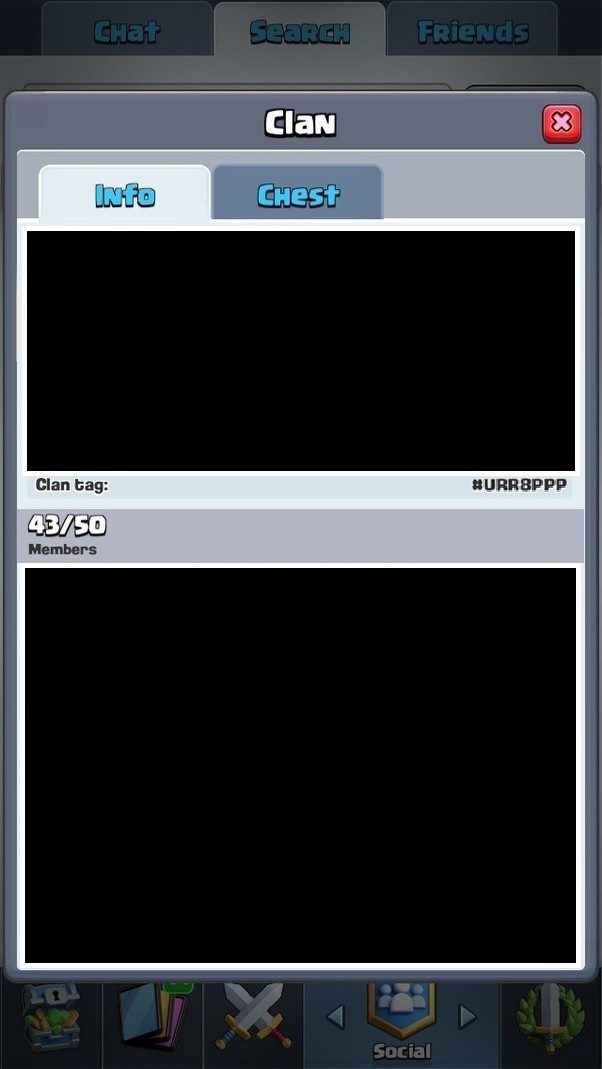
Clash Royale CLAN TAG#URR8PPP
Is Kotlin null safety implemented in compiller?
I wonder if Kotlin somehow need to wrap objects or to carry some flags to support null safety.
Is this information only available during compilation (about null safety) or it is leaked to .class
file or bytecode?
.class
Can I check Kotlin null contract from Java code (for method arguments and return value)?
2 Answers
2
The Kotlin compiler tries really hard to protect from NullPointerExceptions and forces you to handle possible issues appropriately. But even with these very sophisticated means to avoid NPEs there are some situations when it is possible. The best example is when we want to use some java methods in Kotlin. The compiler cannot say if the type (also known as platform type) coming from Java can be null or not. When a value of a platform type is assigned to a variable the compiler allows it but it can lead to the NullPointerException later. That is why Kotlin heavily relies on nullability annotations (@Nullable
and @NotNull
). If these attributes are defined in the Java code which we want to call than the compiler can ensure that no NPE will occur. When the Kotlin compiler generates bytecode it also provides the nullability annotations:
@Nullable
@NotNull
@Nullable
Integer myFunction(@NotNull MyClass first_value, @Nullable String second_value)
This is the way how you can find out about the Kotlin null contract from Java code and as far as I know Kotlin doesn't wrap objects with some additional flag because it would make Kotlin-Java interoperability complicated.
@Nullable
@NotNull
org.jetbrains.annotations
I wonder if Kotlin somehow need to wrap objects or to carry some flags to support null safety.
Just like type safety, null safety pertains to compile time. Not only doesn't Kotlin need to wrap objects, it is the very essence of this feature that it proves at compile time that no runtime checks will be needed.
Is this information only available during compilation (about null safety) or it is leaked to .class
file or bytecode?
.class
The nullability information is available during compilation precisely because it is present in .class
files. Kotlin only compiles your own code, it doesn't compile the entire Standard Library and all your dependencies.
.class
Can I check Kotlin null contract from Java code (for method arguments and return value)?
You can, through reflection (by looking for the nullability annotations). However, as explained above, it would be quite pointless to do so.
null
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I don't see any of the answers (yet) mentioning this. The Kotlin compiler explicitly adds calls to Intrinsics.checkNotNull in the generated .class file. There are currently advanced compiler options so that these are not generated, but there is no conclusive evidence that they do/don't appreciably impact performance. But ultimately, this means it will throw a KotlinNullPointerException if some code didn't get caught during compilation time. Others have described how that can happen.
– Mikezx6r
yesterday