Rewrite Promise-based code with asyncawait
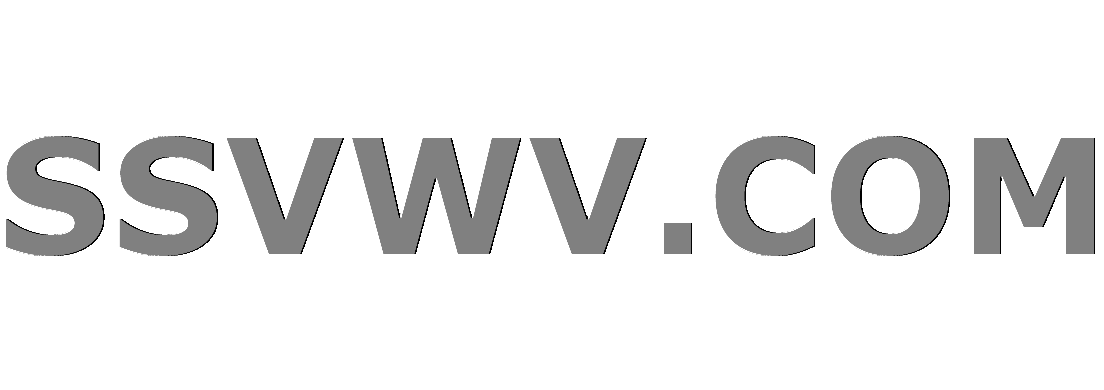
Multi tool use
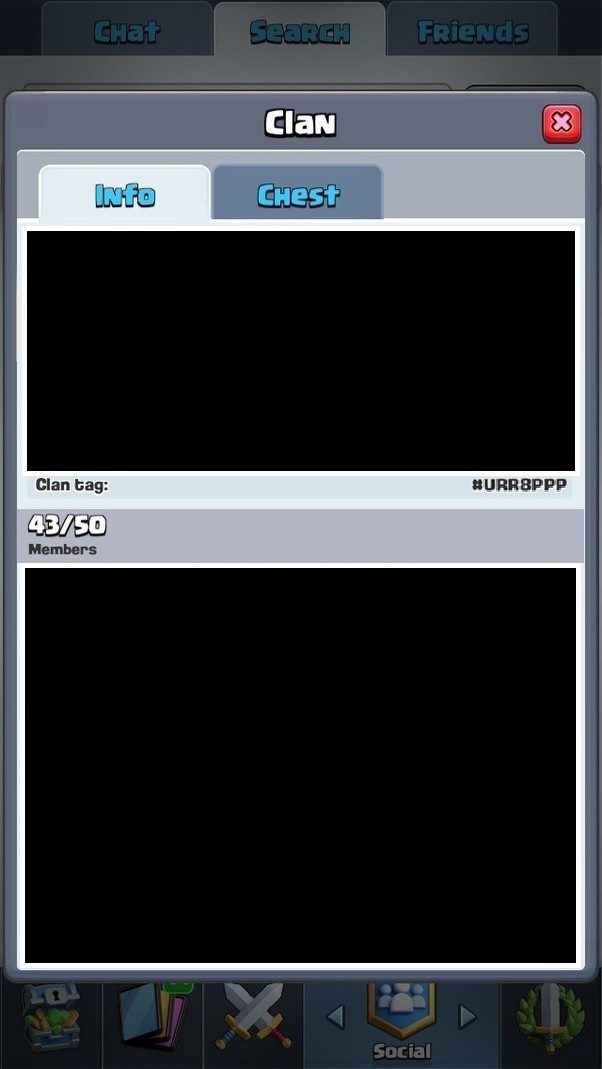

Rewrite Promise-based code with asyncawait
I'm trying to rewrite this Promise
-based code using async
await
:
Promise
async
await
public loadData(id: string): void {
this.loadDataAsync()
.then((data: any): void => {
// Do something with data
})
.catch((ex): void => {
// Do something with ex
});
}
public loadDataAsync(): Promise<any> {
// return Promise somehow
}
The rewritten code I have so far is:
public loadData(id: string): void {
let data: any;
try {
data = await this.loadDataAsync();
} catch(ex) {
// Do something with ex
}
// Do something with data
}
public async loadDataAsync(): Promise<any> {
// return Promise somehow
}
The problem is that I have to make loadData()
method async
since it has await in its body. Then, I have to return some Promise
from it, since async
methods must return a Promise
. But I need to maintain the API of loadData()
method and return void
.
loadData()
async
Promise
async
Promise
loadData()
void
How can I achieve this? How do you break a never ending need for making a method as async
when somewhere deep inside inner calls you're calling an async
method?
async
async
public async loadDataAsync(): Promise<any> {
doesn't make sense. Either use public async loadDataAsync(): any {
or public loadDataAsync(): Promise<any> {
but not both async
and Promise<any>
– 599644
yesterday
public async loadDataAsync(): Promise<any> {
public async loadDataAsync(): any {
public loadDataAsync(): Promise<any> {
async
Promise<any>
@PatrickEvans:
async
methods implicitly return a Promise
and TypeScript requires you to specify so for such method signatures. That's what I've meant by I have to return some Promise from it, since async methods must return a Promise.
– Alexander Abakumov
17 hours ago
async
Promise
I have to return some Promise from it, since async methods must return a Promise.
@599644: You can't specify
any
as a return value type of an async
method in TS. Check this.– Alexander Abakumov
17 hours ago
any
async
1 Answer
1
Ideally if you can change the function declaration, just add the async
keyword to loadData
and use await
inside like so:
async
loadData
await
public async loadData(id: string): void {
let data: any;
try {
data = await this.loadDataAsync();
} catch(ex) {
// Do something with ex
}
}
If you are unable to change the loadData
function declaration, then you can just create an anonymous async
function inside, and then call await
within the anonymous function like so:
loadData
async
await
public loadData(id: string): void {
let data: any;
try {
data = (async () => { return await this.loadDataAsync() })();
} catch(ex) {
// Do something with ex
}
}
public async loadDataAsync(): Promise<any> {
return new Promise((resolve, reject) => {
// Logic goes here
resolve(data);
});
}
Whenever you use the await
keyword, the surrounding function must be marked as async
.
await
async
As was pointed out by the OP in the comments, in TypeScript it is best practice to mark the promise returning method with async
as well (see the loadDataAsync
function above), as this ensures the return type is always a promise. More info on that found here:
async
loadDataAsync
TypeScript Async / Await Tutorial
Regarding your statement
You only use Async when you call Await, not on the promise method.
Could you explain why there is a TS Lint rule promise-function-async
which makes sure all methods returning a Promise
must be async
? From the existence of this rule and its rationale, one might believe marking all Promise
-returning methods with async
is a good practice.– Alexander Abakumov
18 hours ago
You only use Async when you call Await, not on the promise method.
promise-function-async
Promise
async
Promise
async
@AlexanderAbakumov thanks for pointing that out, I updated my answer to reflect that point!
– Asleepace
14 hours ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
async methods automatically return a promise. You return whatever you want inside the async method, that value will be used for the callbacks, meaning what you return isn't what actually gets returned by the function.
– Patrick Evans
yesterday